Adicionando uma Interface com o Usuário
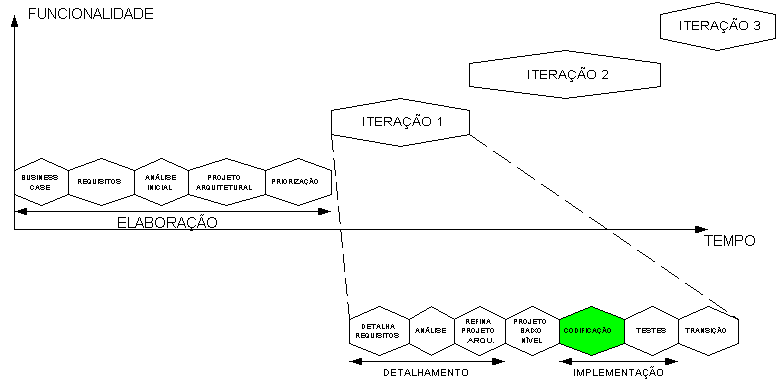
Interface com o usuário
- O business logic estando pronto e testado, podemos fazer
uma interface com o usuário
- Pode-se fazer uma interface em 1 camada, 2 camadas, com applets, 3 camadas com servlets
ou JSP, 4 camadas com RMI, etc.
- Observe que o business logic não muda para qualquer tipo
de interface
- Ao ver o código da interface com o usuário, observe que a lógica é só de
apresentação e de verificação de dados de entrada
- Uma interface Swing em 1 camada pode ser vista aqui
- Está no package tpdvui
- Foi feita com JBuilder 3.0
- A interface parece é a seguinte:
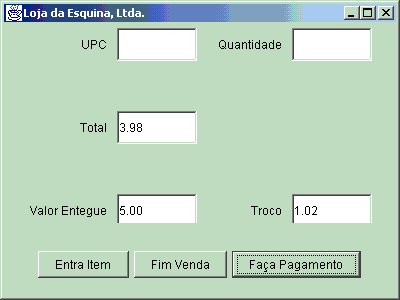
- A interface não é muito funcional e merece ser repensada
Alguns trechos de código
A classe principal
public class Tpdv {
public Tpdv() {
TpdvFrame frame = new TpdvFrame();
// ... um monte de coisinhas
frame.setVisible(true);
}
public static void main(String[] args) {
try {
UIManager.setLookAndFeel(UIManager.getSystemLookAndFeelClassName());
}
catch(Exception e) {
}
new Tpdv();
}
}
A classe TpdvFrame
public class TpdvFrame extends JFrame {
Loja loja = new Loja();
ITPDV tpdv = loja.getTPDV();
// ...
}
Atendimento ao clique do botão Entra Item
- Tem umas 8 linhas de interesse e mais de 20 linhas de verificação e captura de erros
- Isso é perfeitamente normal!
public class TpdvFrame extends JFrame {
// ...
void entraItem_actionPerformed(ActionEvent e) {
if(upc.getText().equals("")) {
JOptionPane.showMessageDialog(this, "UPC deve ser informado");
return;
}
if(quantidade.getText().equals("")) {
JOptionPane.showMessageDialog(this, "Quantidade deve ser informada");
return;
}
int upcInt = 0;
int quant = 0;
try {
upcInt = Integer.parseInt(upc.getText());
} catch(NumberFormatException ex) {
JOptionPane.showMessageDialog(this, "Produto " + upc.getText() + " inexistente");
}
try {
quant = Integer.parseInt(quantidade.getText());
} catch(NumberFormatException ex) {
JOptionPane.showMessageDialog(this, "Quantidade deve ser numérica");
}
try {
tpdv.entraItem(upcInt, quant);
} catch(ProdutoInexistenteException ex) {
JOptionPane.showMessageDialog(this, ex.getMessage());
return;
}
upc.setText("");
quantidade.setText("");
total.setText("");
valorEntregue.setText("");
troco.setText("");
}
// ...
}
Atendimento ao clique do botão Fim Venda
- 2 linhas de interesse e 5 linhas de verificação e captura de erros
public class TpdvFrame extends JFrame {
// ...
void fimVenda_actionPerformed(ActionEvent e) {
try {
tpdv.fimDeVenda();
} catch(NaoHaVendaException ex) {
JOptionPane.showMessageDialog(this, ex.getMessage());
return;
}
total.setText(String.valueOf(tpdv.getVenda().total()));
}
}
Atendimento ao clique do botão Faça Pagamento
- 2 linhas de interesse e 9 linhas de verificação e captura de erros
public class TpdvFrame extends JFrame {
// ...
void façaPagamento_actionPerformed(ActionEvent e) {
if(valorEntregue.getText().equals("")) {
JOptionPane.showMessageDialog(this, "Qual é o valor entregue?");
return;
}
try {
tpdv.façaPagamento((float)Double.parseDouble(valorEntregue.getText()));
} catch(PagamentoInsuficienteException ex) {
JOptionPane.showMessageDialog(this, ex.getMessage());
return;
}
troco.setText(String.valueOf(tpdv.getVenda().getTroco()));
}
}
impl-4 programa anterior