-
LEMBRE DE ESTUDAR O MODELO COMPLETO NO
LIVRO "UML TOOLKIT"
LEMBRE DE ESTUDAR O MODELO COMPLETO NO
LIVRO "UML TOOLKIT"
DEPOIS DE UM BRAINSTORM PARA ACHAR AS
CLASSES DE INTERESSE, CHEGA-SE A:
BorrowerInformation (PARA USAR
UM NOME DIFERENTE DO ATOR), Title, Book Title,
Magazine Title, Item, Reservation, Loan
AS CLASSES SÃO DOCUMENTADAS NUM
DIAGRAMA DE CLASSES JUNTAMENTE COM SEUS RELACIONAMENTOS:
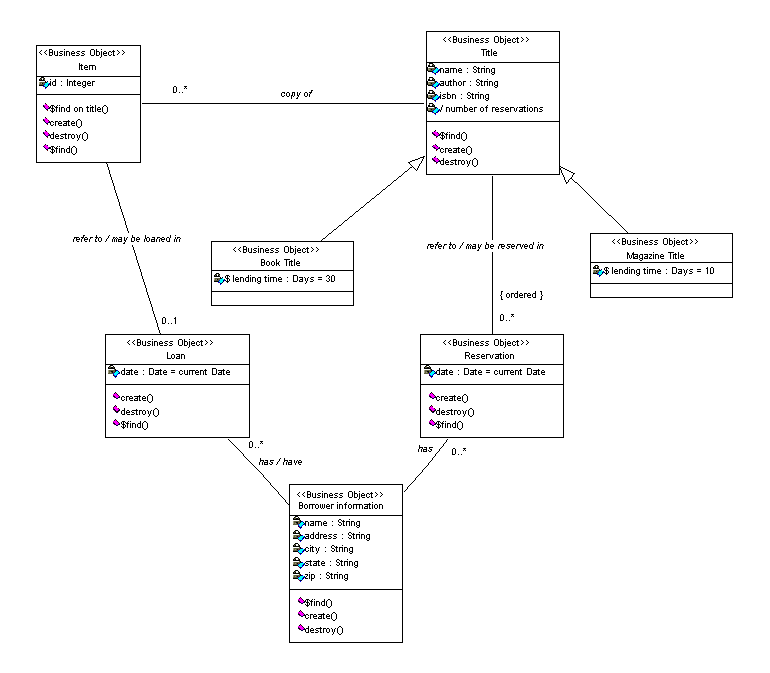
O ESTEREÓTIPO
<<BusinessObject>> INDICA OBJETOS IMPORTANTES
DO DOMÍNIO QUE DEVEM SER ARMAZENADOS PERSISTENTEMENTE
AS OPERAÇÕES E ATRIBUTOS MOSTRADOS
NÃO SÃO FINAIS
REPRESENTAM APENAS AS
RESPONSABILIDADES PRINCIPAIS DAS CLASSES
NENHUMA CLASSE OU OBJETO MERECE SER
OBJETO DE UM DIAGRAMA DE ESTADO AQUI
EMBORA O ARQUIVO .mdl DO EXEMPLO
TENHA USADO UM DIAGRAMA DE ESTADOS SIMPLES PARA
Item E Title
DIAGRAMAS DE SEQUÊNCIA PODEM SER
USADOS, EMBORA AS SITUAÇÕES SEJAM MUITO SIMPLES
EXEMPLO ABAIXO É PARA O USE
CASE DE EMPRÉSTIMO DE UM TÍTULO RESERVADO
AO MODELAR AS INTERAÇÕES,
ESTAMOS AINDA NUM NÍVEL BASTANTE ALTO DE
ABSTRAÇÃO E NÃO FORNECEMOS DETALHES
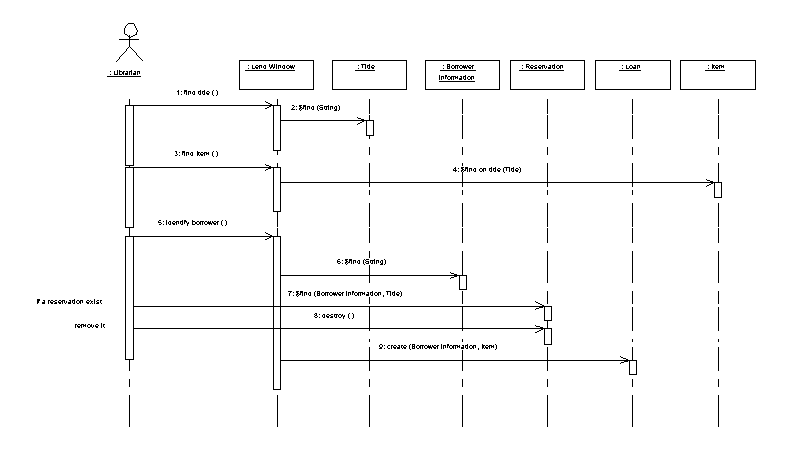
DURANTE A MODELAGEM, FICA APARENTE QUE
PRECISAMOS DE UMA JANELA GUI PARA INTERAGIR E FAZER O
EMPRÉSTIMO
NENHUM DETALHE DA JANELA É DADO
AQUI
SERIA POSSÍVEL ELABORAR UM
POUCO MAIS SOBRE A INTERAFACE COM O USUÁRIO
NESTE PONTO EM DISCUSSÃO COM O USUÁRIO, MAS
DETALHES SÃO FEITOS NA FASE DE PROJETO
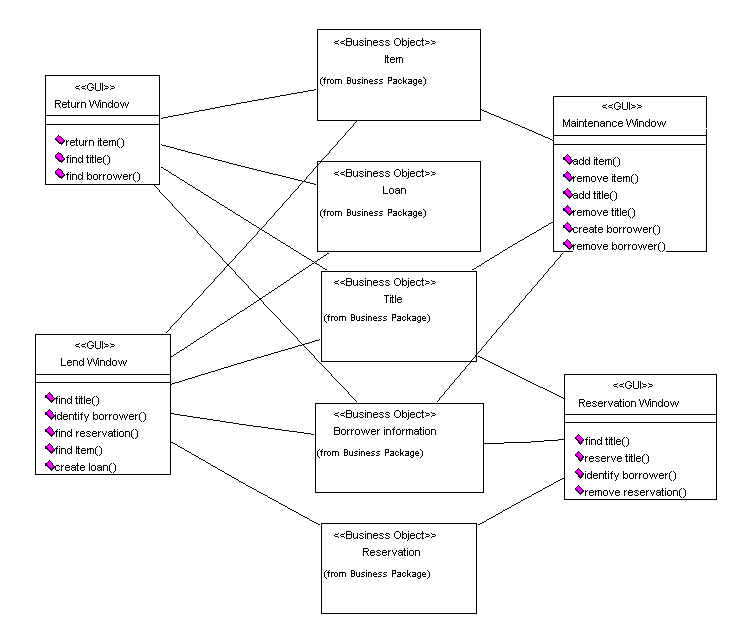
LEMBRE DE ESTUDAR O MODELO COMPLETO NO
LIVRO "UML TOOLKIT"
PROJETO DE ARQUITETURA TAMBÉM É
CHAMADO DE HIGH-LEVEL DESIGN
USANDO O PROCESSO UNIFICADO, A
ARQUITETURA SERÁ INICIALMENTE PLANEJADA MUITO CEDO, JÁ
QUE CADA ITERAÇÃO (MESMO NA ETAPA DE INCEPÇÃO) PASSA
POR ANÁLISE/PROJETO/ETC.
NO PROJETO DA ARQUITETURA, DEFINIMOS OS
PACKAGES (SUBSISTEMAS) INCLUINDO DEPENDÊNCIAS E
MECANISMOS DE COMUNICAÇÃO ENTRE OS PACKAGES
ESTABELECER OS MECANISMOS DE
COMUNICAÇÃO EM MAIS DETALHES É IMPORTANTE JÁ
QUE TIMES DE DESENVOLVIMENTO DIFERENTES ESTARÃO
ENVOLVIDOS EM SUBSISTEMAS DIFERENTES
AO BOLAR A ARQUITETURA:
MANTENHA-A SIMPLES
EVITE DEPENDÊNCIAS
BI-DIRECIONAIS (GERAM ACOPLAMENTO DEMAIS)
SEPARE O APPLICATION LOGIC (OU
BUSINESS LOGIC) QUE TRATA DOS BUSINESS OBJECTS DO
"TECHNICAL LOGIC" QUE SÓ SERVE DE
SUPORTE
ISOLE AS DUAS PARTES
PARA PERMITIR ALTERAR UM SEM AFETAR O
OUTRO MUITO
IDENTIFIQUE A NECESSIDADE DE
USAR BIBLIOTECAS PADRÃO
ISTO É, BIBLIOTECAS
USADAS EM VÁRIOS SUBSISTEMAS
EXEMPLOS: HELP,
ERRO,GUI, DATABASE, COMUNICAÇÃO, ...
NO USE CASE, OS PACKAGES SÃO:
USER INTERFACE PACKAGE
BASEADO NO AWT (DEVERIA
SER SWING, HOJE EM DIA)
COOPERA COM BUSINESS
OBJECTS PACKAGE QUE CONTÉM AS CLASSES
ONDE OS DADOS REALMENTE SÃO ARMAZENADOS
CLASSES DO PACKAGE
"UI" CHAMAM OPERAÇÕES DOS
BUSINESS OBJECTS PARA ACESSAR E ALTERAR
OS DADOS
BUSINESS OBJECTS PACKAGE
INCLUI CLASSES
IDENTIFICADAS DURANTE A ANÁLISE
AQUI, DEVEMOS COMPLETAR
TODAS AS OPERAÇÕES E ADICIONAR SUPORTE
PARA PERSISTÊNCIA
HÁ COOPERAÇÃO COM O
DATABASE PACKAGE, NO SENTIDO DE QUE TODOS
OS OBJETOS NO BUSINESS OBJECTS PACKAGE
DEVEM HERDAR DA CLASSE
"Persistent" DO DATABASE
PACKAGE
DATABASE PACKAGE
FORNECE PERSISTÊNCIA
PARA CLASSES DO BUSINESS OBJECTS PACKAGE
NA VERSÃO DO SOFTWARE
CONTIDA NO EXEMPLO, OS OBJETOS SÃO
ARMAZENADOS EM ARQUIVOS
UTILITY PACKAGE
CONTÉM SERVIÇOS USADOS
POR OUTROS PACKAGES
POR ENQUANTO, SÓ
CONTÉM A CLASSE ObjId USADA PARA
REFERENCIAR OBJETOS PERSISTENTES
USADO NOS OUTROS
3 PACKAGES
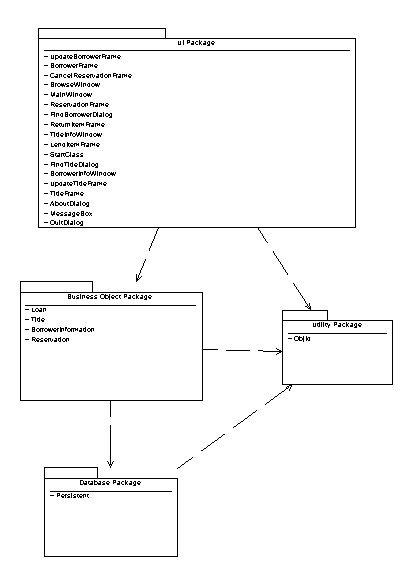
AGORA, PODEMOS PASSAR PARA O LOW-LEVEL
DESIGN (OU DETAILED DESIGN)
ENVOLVE O REFINAMENTO DOS
DIAGRAMAS PARA OFERECER MAIS DETALHES VISANDO SE
APROXIMAR DE UM MODELO CODIFICÁVEL
OBJETOS DEVEM SER ARMAZENADOS
PERSISTENTEMENTE
CAMADA DE BANCO DE DADOS
ADICIONADA PARA PRESTAR ESTE SERVIÇO
ALTERNATIVAS
BANCO DE DADOS OO
BANCO DE DADOS RELACIONAL COM
CAMADA DE TRADUÇÃO OBJECT-TO-TABLE
O EXEMPLO USA ARQUIVOS E NÃO
UIM BANCO DE DADOS PORQUE É UM BRINQUEDO
EXERCÍCIO: ALTERE O
EXEMPLO PARA USAR JDBC (COM JAVABEANS)
DETALHES SÃO ESCONDIDOS
AS OPERAÇÕES SÃO store(),
update(), (delete(), find(), ETC.
TODA PERSISTÊNCIA É IMPLEMENTADA NA
CLASSE Persistent QUE OBJETOS PERSISTENTES DEVEM HERDAR
AS SUB-CLASSE DEVEM FORNECER
MÉTODOS read() E write()
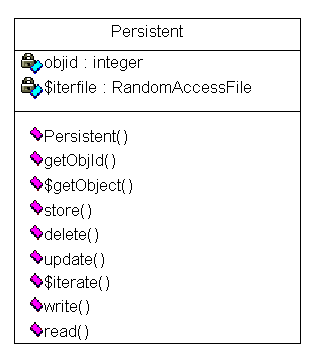
A CLASSE ObjId É USADA PARA OFERECER
REFERÊNCIAS A OBJETOS PERSISTENTES
A REFERÊNCIA É A MESMA QUE O
OBJETO ESTEJA NA MEMÓRIA OU NO DISCO
A OPERAÇÃO
Persistent.getObject() PODE RECEBER UM ObjId E
RETORNAR O OBJETO
getObject() NORMALMENTE
ENVOLVE POLIMORFISMO PARA QUE CADA CLASSE
PERSISTENTE POSSA VERIFICAR TIPOS E FAZER
AS CONVERSÕES NECESSÁRIAS
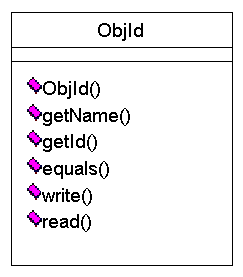
NA IMPLEMENTAÇÃO ATUAL, A
PERSISTÊNCIA PODE SERE MELHORADA
NUM update(), A CÓPIA ANTIGA É
ABANDONADA E UMA NOVA CÓPIA É GRAVADA NO FIM DO
ARQUIVO
MODELO SEMELHANTE AO MODELO DE ANÁLISE,
COM MAIS DETALHES
EXEMPLO: O FATO DE QUE HERDAM DA
CLASSE Persistente
ALGUMAS OPERAÇÕES MUDARAM DE
NOME
OUTRAS OPERAÇÃO FORAM
DESDOBRADAS EM VÁRIAS OPERAÇÕES
ALGUMAS MUDANÇAS QUE OCORRERAM NO
EXEMPLO
ATRIBUTO date NÃO FOI
IMPLEMENTADO NAS CLASSES Loan E Reservation, JÁ
QUE NÃO TEMOS SUPORTE À VERIFICAÇÃO DO PRAZO
DE DEVOLUÇÃO (NESTA ITERAÇÃO)
JÁ QUE A ÚNICA DIFERENÇA
ENTRE LIVROS E REVISTAS É O PRAZO DE DEVOLUÇÃO
E JÁ QUE ESTE PRAZO NÃO FOI IMPLEMENTADO, O
PROJETO SIMPLIFICOU A ANÁLISE E REMOVEU AS
SUBCLASSES, SUBSTITUINDO-A POR UM ATRIBUTO DE
TIPO (LIVRO VERSUS REVISTA)
CUIDADO! EU NÃO
CONCORDO COM OS AUTORES! ELES NÃO
DEVERIAM SUBSTITUIR HERANÇA POR
ATRIBUTOS DE TIPO
ASSINATURAS COMPLETAS DEVEM SER USADAS
NAS OPERAÇÕES
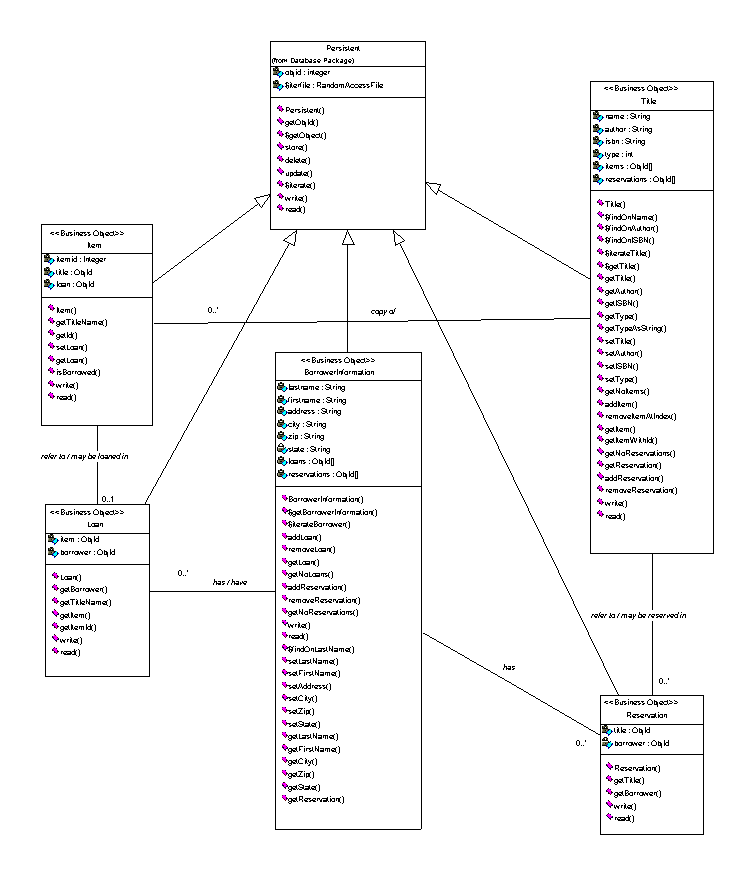
FICAM "EM CIMA" DOS OUTROS
PACKAGES
REPRESENTA OS SERVIÇOS E INFORMAÇÃO
QUE O SISTEMA OFERECE AO USUÁRIO
OS MODELOS DINÂMICOS (SEQUÊNCIA, ETC)
SÃO FREQUENTEMENTE COLOCADOS AQUI JÁ QUE O INÍCIO DA
SEQUÊNCIA NORMALMENTE É UMA INTERAÇÃO COM O USUÁRIO
EXEMPLO: SEQUÊNCIA PARA USE CASE Add
Title
OBSERVE O NÍVEL DE
DETALHAMENTO, INCLUINDO ASSINATURAS COMPLETAS
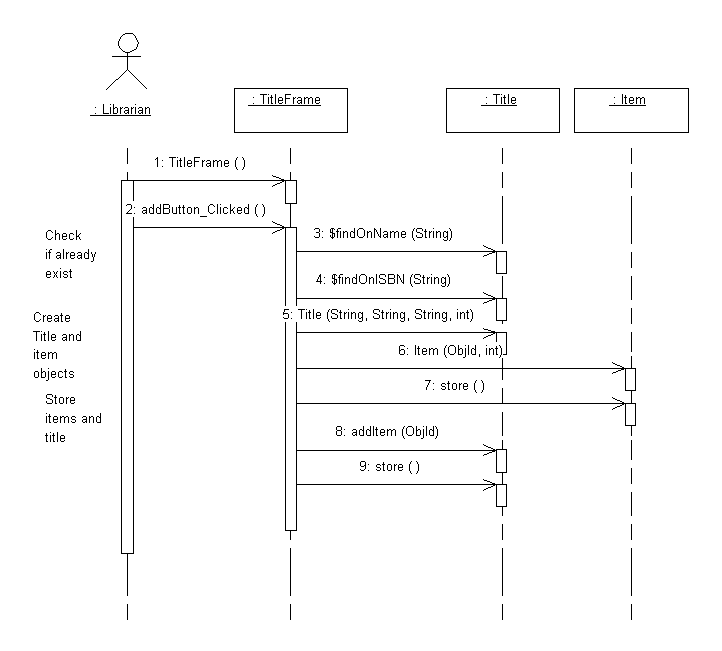
INICIA-SE O PROJETO DA INTERFACE COM O
USUÁRIO (UI) NA FASE DE ANÁLISE
COMPLETA NA FASE DE PROJETO
O UI É BASEADA NOS USE CASES E É
SEPARADA EM SEÇÕES, PARA REFLETIR AS OPÇÕES DE MENU
EXEMPLO: Functions, Information,
Maintenance
LAYOUT É FEITO COM UM INTERACTIVE
DEVELOPMENT ENVIRONMENT (IDE)
DELPHI, VISUAL BASIC, J BUILDER,
VISUAL CAFE, ...
O CÓDIGO DA UI É GERADO PELO
IDE
FOCO NA LINGUAGEM, NÃO MAIS EM PROJETAR
A SOLUÇÃO
MINHA OPINIÃO É QUE USAR DIAGRAMAS DE
COMPONENTES PARA MANTER CONTROLE DO CÓDIGO FONTE NÃO É
ÚTIL
MELHOR USAR O PRÓPRIO IDE, E/OU
PACOTES DE CONTROLE DE VERSÃO DE CÓDIGO FONTE
LEMBRE QUE O PROCESSO É ITERATIVO E QUE
MUITOS PROBLEMAS SERÃO DESCOBERTOS DURANTE A
CODIFICAÇÃO
MANTENHA OS MODELOS (ANÁLISE,
PROJETO, CÓDIGO) SINCRONIZADOS!
EXAMINE O CÓDIGO DA APLICAÇÃO-EXEMPLO
NO CDROM DO LIVRO "UML TOOLKIT"
EXEMPLO: A CLASSE Loan
//
// Unified Library Application
// Case study in Unified Modeling Language Toolkit
//
// Loan.java: represents a loan. The loan refer to one
// title and one borrower.
//
// Copyright (c) 1998 John Wiley & Sons, Inc. All rights reserved.
// Reproduction or translations of this work beyond that permitted
// in Section 117 of the 1976 United States Copyright Act without
// the express written permission of the copyright owner is unlawful.
// Requests for further information should be addressed to Permissions
// Department, John Wiley & Sons, Inc. The purchaser may make back-up
// copies for his/her own use only and not for distribution or resale.
// The Publisher assumes no responsibility for errors, omissions, or
// damages, caused by the use of these programs of from the use of the
// information contained herein.
package bo;
import util.ObjId;
import db.*;
import java.io.*;
import java.util.*;
public class Loan extends Persistent {
private ObjId item;
private ObjId borrower;
public Loan(){
}
public Loan(ObjId it, ObjId b) {
item = it;
borrower = b;
}
public BorrowerInformation getBorrower() {
BorrowerInformation ret =
(BorrowerInformation) Persistent.getObject(borrower);
return ret;
}
public String getTitleName() {
Item it = (Item) Persistent.getObject(item);
return it.getTitleName();
}
public Item getItem() {
Item it = (Item) Persistent.getObject(item);
return it;
}
public int getItemId() {
Item it = (Item) Persistent.getObject(item);
return it.getId();
}
public void write(RandomAccessFile out)
throws IOException {
item.write(out);
borrower.write(out);
}
public void read(RandomAccessFile in)
throws IOException {
item = new ObjId();
item.read(in);
borrower = new ObjId();
borrower.read(in);
}
}
caso3.htm programa anterior